12.5 Area Lights
Area lights are light sources defined by one or more Shapes that emit light from their surface, with some directional distribution of radiance at each point on the surface. In general, computing radiometric quantities related to area lights requires computing integrals over the surface of the light that often can’t be computed in closed form. This issue is addressed with the Monte Carlo integration techniques in Section 14.2. The reward for this complexity (and computational expense) is soft shadows and more realistic lighting effects, rather than the hard shadows and stark lighting that come from point lights. (See Figure 12.16.)
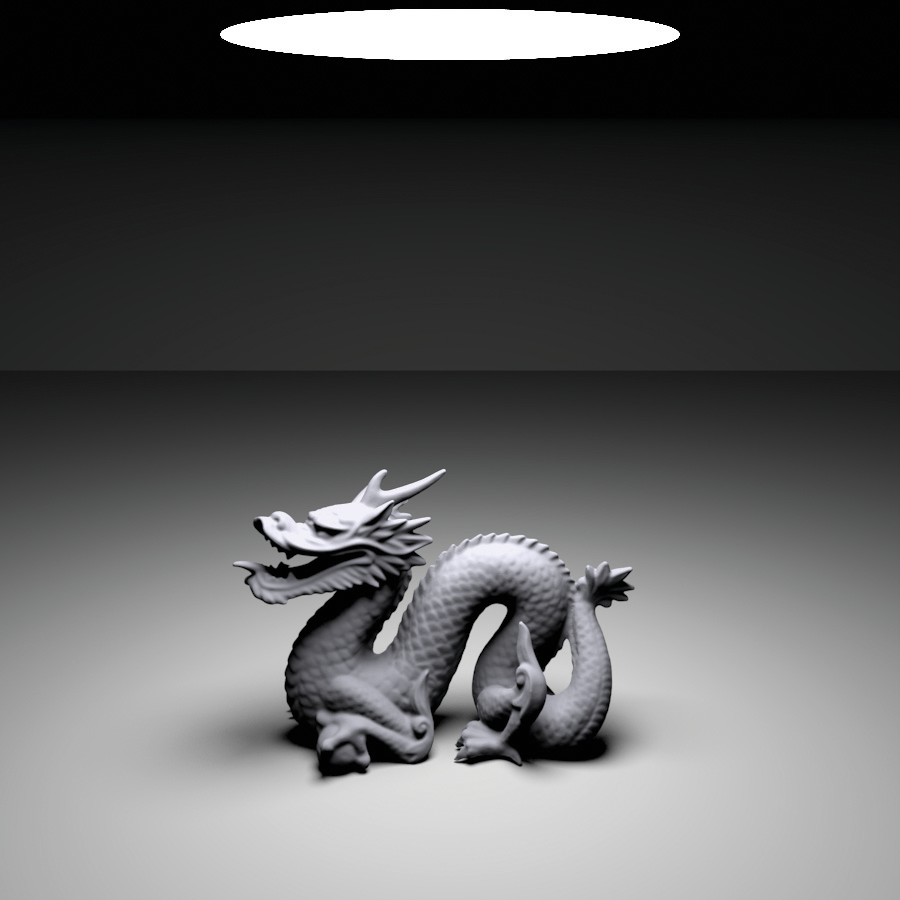
Figure 12.17 shows the effect of varying the size of an area light source used to illuminate the dragon; compare its soft look to illumination from a point light in Figure 12.6.
The AreaLight class is an abstract base class that inherits from Light. Implementations of area lights should inherit from it.
AreaLight adds a single new method to the general Light interface, AreaLight::L(). Implementations are given a point on the surface of the light represented by an Interaction and should evaluate the area light’s emitted radiance, , in the given outgoing direction.
For convenience, there is a method in the SurfaceInteraction class that makes it easy to compute the emitted radiance at a surface point intersected by a ray.
DiffuseAreaLight implements a basic area light source with a uniform spatial and directional radiance distribution. The surface it emits from is defined by a Shape. It only emits light on the side of the surface with outward-facing surface normal; there is no emission from the other side. (The Shape::reverseOrientation value can be set to true to cause the light to be emitted from the other side of the surface instead.) DiffuseAreaLight is defined in the files lights/diffuse.h and lights/diffuse.cpp.
Because this area light implementation emits light from only one side of the shape’s surface, its L() method just makes sure that the outgoing direction lies in the same hemisphere as the normal.
The DiffuseAreaLight::Sample_Li() method isn’t as straightforward as it has been for the other light sources described so far. Specifically, at each point in the scene, radiance from area lights can be incident from many directions, not just a single direction as was the case for the other lights (Figure 12.18). This leads to the question of which direction should be chosen for this method. We will defer answering this question and providing an implementation of this method until Section 14.2, after Monte Carlo integration has been introduced.
Emitted power from an area light with uniform emitted radiance over the surface can be directly computed in closed form: