7.6 Maximized Minimal Distance Sampler
The -sequence sampler is more effective than the stratified sampler, thanks to being stratified over all elementary intervals. However, it still sometimes generates sample points that are close together. An alternative is to use a different pair of generator matrices that not only generate -sequences but that are also specially designed to maximize the distance between samples; this approach is implemented by the MaxMinDistSampler. (See the “Further Reading” section for more details about the origin of these generator matrices.)
There are 17 of these specialized matrices, one for each power-of-two number of samples up to samples; a pointer to the appropriate one is stored in CPixel in the constructor.
Figure 7.32 shows a few of these matrices.
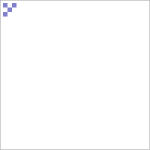
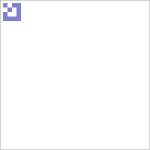
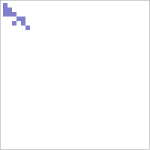
Figure 7.33 shows the points that one of the matrices generates. Note that the same sampling pattern is used in each of the pixels shown there; when the matrices were found, distance between sample points was evaluated using toroidal topology—as if the unit square was rolled into a torus—to allow for high-quality sample tiling.
The MaxMinDistSampler uses the generator matrix to compute the pixel samples. The first 2D sample dimension’s value is set by uniformly stepping in the first dimension and the second comes from the generator matrix.
The remaining dimensions are sampled using the first two Sobol matrices, like the ZeroTwoSequenceSampler. We have found slightly better results with this approach (versus using the CMaxMinDist matrices) for samples in non-image dimensions of the sample vector. Therefore, the corresponding fragment <<Generate remaining samples for MaxMinDistSampler>> isn’t included here.